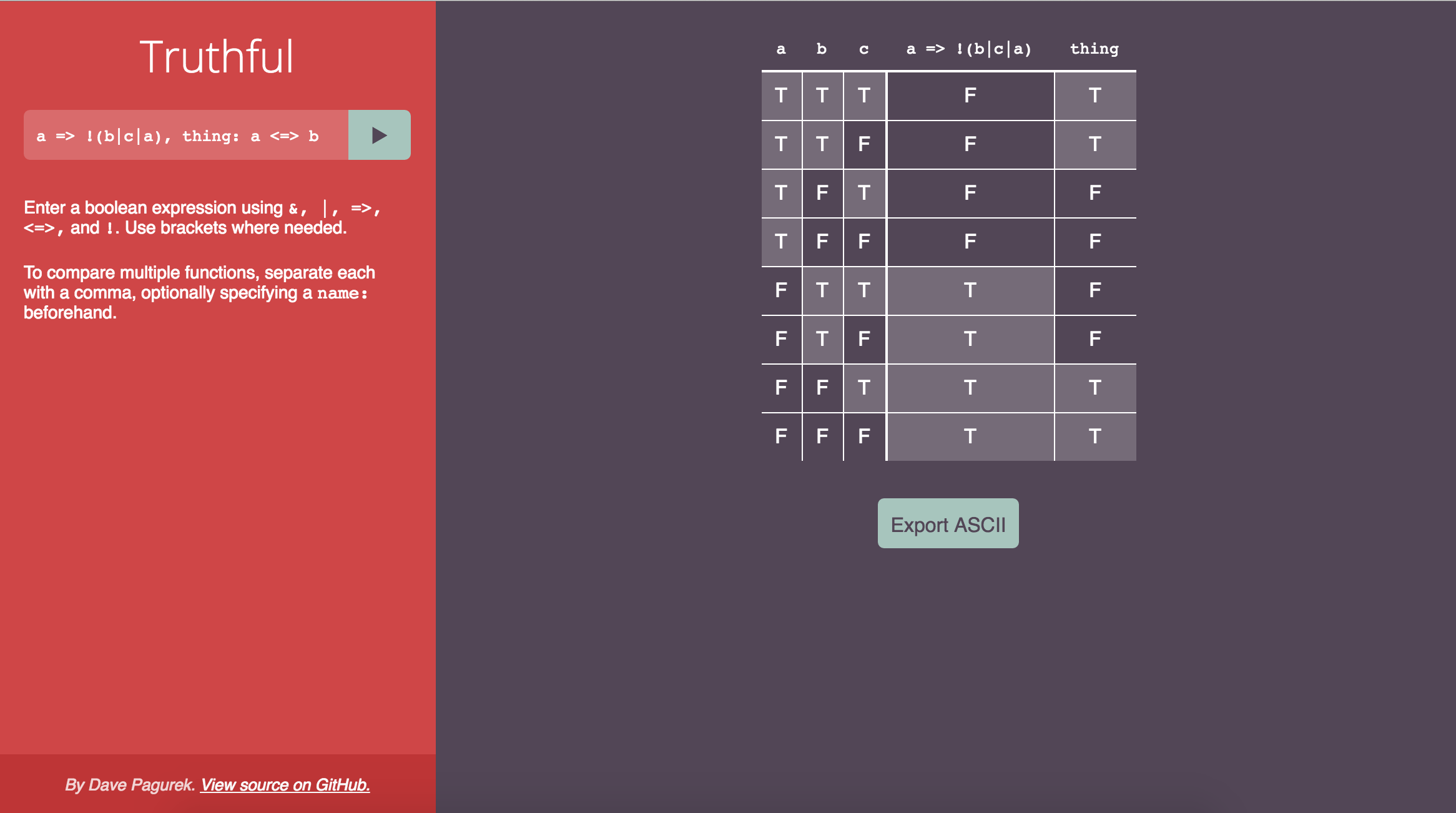
Truthful
In many circuits and formal logic courses, it's useful to be able to quickly see what a truth table for a Boolean expression is. I made a parser and evaluator tool to make that process simpler.
This is a tool to enter boolean expressions and generate truth tables.
Using Truthful.js
Fork the repo and set up dependencies:
npm install
npm install -g bower
bower install
Include the Javascript file in your HTML:
<script src="Truthful.js"></script>
...or in Node:
var Truthful = require("./js/Truthful.js");
Expressions
Create an algebraic expression like this:
var expression = Truthful.expression("!(b|c|a)");
Expressions can use & for AND, | for OR, => for IMPLIES, <=> for IFF, ! for NOT and round brackets for grouping. Literals true and false may also be used.
The precedence of operators is () > ! > & > | > => > <=>.
To get the result of an expression, pass in an object with values for each variable:
Truthful.expression("c&(a|b)").result({
"a": true,
"b": false,
"c": true,
})
// Returns true
If a variable's value is not specified, it defaults to false.
Expressions can return an object with keys for the variables present in it (rather than an array, so that each variable only can occur once):
Truthful.expression("a|b|c").variables()
// Returns {a:true,b:true,c:true}
Expressions can also render string versions of themselves with brackets specifying order of operations explicitly:
Truthful.expression("a|b&(!c)").string()
// Returns "(a|(b&(!c)))"
Truth Tables
Get an HTML <table> element of a truth table by passing an input string:
var table = Truthful.truthTable("a => !(b|c|a)");
document.body.appendChild(table.html());
A string containing an ASCII table formatted for George can also be returned:
Truthful.truthTable("a|b").george()
/*
Returns a string containing a table such as:
a | b || a|b
T | T || T
T | F || T
F | T || T
F | F || F
*/
To compare multiple functions, separate each with a comma. A function can be named by optionally specifying a name: beforehand:
Truthful.truthTable("a => !(b|c|a), thing: a <=> b").george()
/*
Returns
a | b | c || a => !(b|c|a) | thing
T | T | T || F | T
T | T | F || F | T
T | F | T || F | F
T | F | F || F | F
F | T | T || T | F
F | T | F || T | F
F | F | T || T | T
F | F | F || T | T
*/
Error handling
If there is an error with user input, Truthful will throw a new Error() with a useful message. If using Truthful for arbitrary user data, it is recommended to parse input in a try { } catch (err) { } and show the user err.message.
Contributing
- Make an issue on GitHub if you notice a broken feature
- Fork the repo and make a branch for any updates
- After cloning locally, run npm install
- Add tests for new features
- Run tests with npm test
- Create a pull request
For more details, see GitHub's contribution guide.